React has taken the web development world by storm, and for good reason! 🚀 Did you know that over 40% of developers use React as their primary web framework? Whether you're a coding newbie or a seasoned dev looking to level up, mastering React is a game-changer. In this guide, we'll break down the essential concepts that'll have you building awesome web apps in no time. Ready to React-ify your skills? Let's dive in!
1. The Building Blocks: Components and JSX
At the heart of every React application lies two fundamental concepts: Components and JSX. Let's unpack these powerful tools that make React so intuitive and efficient.
Components: The LEGO Bricks of React
Imagine you're building a LEGO castle. Each brick represents a component in React. Just as you can reuse LEGO bricks to create different structures, React components are reusable pieces of code that define a part of your user interface.
Here's what makes components so awesome:
- Reusability: Write once, use everywhere. Need a button? Create a Button component and use it all over your app!
- Maintainability: Changes to a component automatically update everywhere it's used. Talk about efficiency!
- Modularity: Break down complex UIs into manageable, bite-sized pieces. It's like dividing a big problem into smaller, solvable chunks.
In modern React, we primarily use functional components. They're just JavaScript functions that return JSX. Here's a simple example:
function Welcome(props) { return <h1>Hello, {props.name}!</h1>; }
JSX: HTML's Cool Cousin
Now, let's talk about JSX. It's like HTML took a JavaScript vacation and came back with superpowers! JSX allows you to write HTML-like code right in your JavaScript files. It's what makes React feel so natural for web developers.
Key things to remember about JSX:
- It's not actually HTML: JSX gets transformed into JavaScript behind the scenes.
- Camel Case Attributes: In JSX, we use
className
instead ofclass
,onClick
instead ofonclick
, etc. - Expressions in Curly Braces: Want to embed JavaScript expressions? Just wrap them in curly braces!
Here's JSX in action:
const element = <h1>Welcome to {user.name}'s Profile!</h1>;
Putting It All Together
When you combine components and JSX, you get a powerful system for building user interfaces. You can nest components, pass data between them, and create complex UIs with ease.
function App() { return ( <div> <Welcome name="Alice" /> <Welcome name="Bob" /> <Welcome name="Charlie" /> </div> ); }
In this example, we're using our Welcome
component three times, each with a different name. This showcases the reusability and composability of React components.
By mastering components and JSX, you're laying a solid foundation for your React journey. These concepts are the building blocks you'll use to create everything from simple widgets to complex web applications.
2. Passing Data: Props and the Magic of Reusability
Now that we've got our LEGO bricks (components) and our special glue (JSX), it's time to learn how to pass information between these components. Enter props: the data superhighway of React!
What Are Props?
Props, short for properties, are how we pass data from a parent component to a child component. Think of them as arguments to a function. Just as you can customize a function's behavior by passing different arguments, you can customize a component's output by passing different props.
Here's a simple example:
function Greeting(props) { return <h1>Hello, {props.name}!</h1>; } function App() { return ( <div> <Greeting name="Alice" /> <Greeting name="Bob" /> </div> ); }
In this example, name
is a prop we're passing to the Greeting
component. The Greeting
component then uses this prop to customize its output.
The Power of Reusability
Props are the secret sauce that makes React components truly reusable. By passing different props, we can use the same component in various contexts throughout our application. This "write once, use everywhere" approach is a game-changer for productivity and maintainability.
Let's look at a more complex example:
function UserCard(props) { return ( <div className="user-card"> <img src={props.avatar} alt={props.name} /> <h2>{props.name}</h2> <p>Age: {props.age}</p> <p>Role: {props.role}</p> </div> ); } function App() { return ( <div> <UserCard name="Alice Johnson" age={28} role="Developer" avatar="https://example.com/alice.jpg" /> <UserCard name="Bob Smith" age={35} role="Designer" avatar="https://example.com/bob.jpg" /> </div> ); }
Here, our UserCard
component is reusable and flexible. We can create cards for different users just by passing different props.
The Special "children" Prop
React has a special prop called children
. This prop allows you to pass components as data to other components, enabling component composition.
function Layout(props) { return ( <div className="layout"> <header>My Awesome App</header> <main>{props.children}</main> <footer>© 2025 My Awesome App</footer> </div> ); } function App() { return ( <Layout> <h1>Welcome to My App!</h1> <p>This content is passed as the children prop.</p> </Layout> ); }
In this example, everything between the <Layout>
tags becomes the children
prop of the Layout
component. This pattern is incredibly powerful for creating reusable wrapper components.
Best Practices for Props
- Keep components pure: Treat props as read-only. Don't try to modify them within the component.
- Use default props: For non-required props, set default values to make your components more robust.
- Validate your props: Use PropTypes (or TypeScript) to catch bugs early by validating the types of props.
import PropTypes from 'prop-types'; function Greeting({ name = "Guest" }) { return <h1>Hello, {name}!</h1>; } Greeting.propTypes = { name: PropTypes.string };
Conclusion
Props are the lifeblood of React applications, allowing data to flow through your component tree and enabling true component reusability. By mastering props, you're well on your way to creating flexible, maintainable React applications.
3. State Management: The Heart of React Applications
If props are the veins carrying data through your React app, then state is its beating heart. State is what brings your components to life, allowing them to remember information, respond to user actions, and update dynamically. Let's dive into this crucial concept!
What is State?
State represents the internal data of a component that can change over time. Unlike props, which are passed down from parent components, state is managed within the component itself. When state changes, React efficiently re-renders the component to reflect these changes.
Enter the useState Hook
In modern React, we manage state in functional components using the useState
hook. It's like giving your component a memory!
Here's a simple example:
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount(count + 1)}> Click me </button> </div> ); }
Let's break this down:
- We import
useState
from React. useState(0)
initializes our state with a value of 0.- It returns an array with two elements: the current state value (
count
) and a function to update it (setCount
). - When the button is clicked, we call
setCount
with the new value, triggering a re-render.
State Updates and Re-renders
When you call the state updating function (like setCount
in our example), React schedules a re-render of your component. This is how the UI stays in sync with your data.
However, state updates may be batched for performance reasons. If you need to update state based on the previous state, use the functional form:
setCount(prevCount => prevCount + 1);
This ensures you're always working with the most up-to-date state value.
Complex State with Objects
State doesn't have to be just numbers or strings. It can be objects or arrays too. When dealing with complex state, remember to spread your previous state to avoid losing data:
const [user, setUser] = useState({ name: 'Alice', age: 28 }); // Updating only the age setUser(prevUser => ({ ...prevUser, age: 29 }));
Other Essential Hooks
While useState
is your bread and butter, React provides several other hooks to handle different scenarios:
- useEffect: For performing side effects in your components.
useEffect(() => { document.title = `You clicked ${count} times`; }, [count]); // Only re-run the effect if count changes
- useContext: For consuming context in your components.
const theme = useContext(ThemeContext);
- useRef: For persisting values across renders without causing re-renders.
const inputRef = useRef(null); // Later: inputRef.current.focus();
- useMemo and useCallback: For optimizing performance by memoizing values and callbacks.
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
Custom Hooks: Reusing Stateful Logic
One of the most powerful features of hooks is the ability to create your own! Custom hooks allow you to extract component logic into reusable functions.
function useWindowWidth() { const [width, setWidth] = useState(window.innerWidth); useEffect(() => { const handleResize = () => setWidth(window.innerWidth); window.addEventListener('resize', handleResize); return () => window.removeEventListener('resize', handleResize); }, []); return width; } // Usage in a component function MyComponent() { const width = useWindowWidth(); return <div>Window width is {width}</div>; }
This custom hook encapsulates all the logic for tracking the window width, making it reusable across multiple components.
State Management Best Practices
- Keep it local: Manage state as close to where it's used as possible.
- Lift it up: If multiple components need the same state, lift it to their closest common ancestor.
- Keep it flat: Avoid deeply nested state objects for easier updates and better performance.
- Use context wisely: For global state that many components need, consider using React Context along with hooks.
State management is a vast topic, and as your apps grow, you might explore libraries like Redux or Recoil. But for most applications, React's built-in state management capabilities are more than sufficient.
4. Dynamic Rendering: Keys and Virtual DOM
Now that we've got our components built and our state managed, it's time to dive into how React efficiently updates the UI. This is where the magic of React's rendering process, the importance of keys, and the power of the Virtual DOM come into play.
The React Rendering Process
When you're building a React app, you're not directly manipulating the DOM (Document Object Model). Instead, you're describing what you want the UI to look like at any given moment. React takes care of updating the DOM to match your description.
Here's a simplified version of what happens:
- Your component's state or props change.
- React re-renders your component (calls your component function again).
- React compares the new output with the previous one.
- React updates only the parts of the DOM that have changed.
This process is incredibly efficient, thanks to two key concepts: the Virtual DOM and reconciliation.
The Virtual DOM: React's Performance Secret
The Virtual DOM is a lightweight copy of the actual DOM that React keeps in memory. When your component re-renders, React first updates this Virtual DOM, which is much faster than updating the real DOM.
Once the Virtual DOM is updated, React compares it with a snapshot of the previous Virtual DOM (a process called "diffing"). It then calculates the most efficient way to update the real DOM, minimizing the number of actual DOM manipulations.
This process happens so quickly that users perceive the updates as instantaneous, even for complex UIs!
Keys: The Unsung Heroes of Efficient Rendering
When rendering lists of elements in React, you'll often see a key
prop being used. Keys play a crucial role in React's reconciliation process, especially when dealing with dynamic lists.
function TodoList({ todos }) { return ( <ul> {todos.map(todo => ( <li key={todo.id}>{todo.text}</li> ))} </ul> ); }
Here's why keys are so important:
- Unique Identification: Keys help React identify which items have changed, been added, or been removed in a list.
- Efficient Updates: With keys, React can update only the elements that have changed, rather than re-rendering the entire list.
- Maintaining State: Keys ensure that component state is preserved even when the position of an element changes within the list.
Best Practices for Keys
- Use Stable IDs: Ideally, use unique and stable identifiers from your data as keys (like database IDs).
- Avoid Index as Key: While tempting, using the array index as a key can lead to issues with component state and performance in dynamic lists.
- Keep Keys Unique Within Siblings: Keys only need to be unique among siblings, not globally.
Optimizing Renders with React.memo
Sometimes, you might want to prevent a component from re-rendering unless its props have changed. React.memo is a higher-order component that can help with this:
const MemoizedComponent = React.memo(function MyComponent(props) { /* render using props */ });
React.memo
does a shallow comparison of props. If your component renders the same result given the same props, wrapping it in React.memo
can lead to performance boosts.
The Rule of Purity in Rendering
React components should be pure functions with respect to their props and state. This means:
- Given the same props and state, a component should always render the same output.
- The render process shouldn't have side effects (like modifying global variables or making API calls).
Side effects should be handled in event handlers or hooks like useEffect
.
Debugging Render Issues
React DevTools is your best friend when it comes to understanding and optimizing your component's render behavior. It can help you:
- Visualize component re-renders.
- Identify unnecessary re-renders.
- Understand the props and state that are triggering re-renders.
Remember, while React's rendering process is highly optimized, it's still possible to create performance issues with complex or poorly structured components. Always strive for simplicity and follow React's best practices for optimal performance.
5. Advanced Concepts: Context, Portals, and Error Boundaries
As you become more comfortable with React's core concepts, it's time to explore some advanced features that can take your applications to the next level. Let's dive into Context, Portals, and Error Boundaries.
Context: Solving the Prop Drilling Problem
As your React application grows, you might find yourself passing props through multiple levels of components. This is often called "prop drilling" and can make your code harder to maintain. Context provides a way to share values like these between components without explicitly passing a prop through every level of the tree.
Here's how to use Context:
- Create a context:
const ThemeContext = React.createContext('light');
- Provide a context value:
function App() { return ( <ThemeContext.Provider value="dark"> <Toolbar /> </ThemeContext.Provider> ); }
- Consume the context value:
function ThemedButton() { const theme = useContext(ThemeContext); return <Button theme={theme} />; }
Context is great for sharing global data like user authentication, theme preferences, or language settings.
Portals: Rendering Outside the DOM Hierarchy
Sometimes, you need to render a child component outside of its parent DOM hierarchy. This is where Portals come in handy. Portals are commonly used for modals, tooltips, or floating menus.
Here's a simple example of a Portal:
import ReactDOM from 'react-dom'; function Modal({ children }) { return ReactDOM.createPortal( children, document.getElementById('modal-root') ); } function App() { return ( <div> <h1>My App</h1> <Modal> <h2>This is a modal</h2> <p>It's rendered outside the DOM hierarchy of the parent component.</p> </Modal> </div> ); }
In this example, the Modal component will be rendered in the 'modal-root' element, regardless of where it's called in the component tree.
Error Boundaries: Graceful Error Handling
In a perfect world, our applications would never crash. But in reality, errors happen. Error Boundaries in React provide a way to catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of the component tree that crashed.
Here's how to create an Error Boundary:
class ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false }; } static getDerivedStateFromError(error) { return { hasError: true }; } componentDidCatch(error, errorInfo) { logErrorToMyService(error, errorInfo); } render() { if (this.state.hasError) { return <h1>Something went wrong.</h1>; } return this.props.children; } } // Usage <ErrorBoundary> <MyComponent /> </ErrorBoundary>
Error Boundaries are class components that implement either getDerivedStateFromError()
or componentDidCatch()
(or both). They're incredibly useful for improving user experience by preventing the entire app from crashing due to an error in one component.
Suspense: Handling Async Operations Gracefully
React Suspense is a feature that allows you to "suspend" rendering of a component while it's waiting for something (like data fetching). It's particularly useful for handling loading states in your application.
Here's a basic example:
import React, { Suspense } from 'react'; const LazyComponent = React.lazy(() => import('./LazyComponent')); function MyComponent() { return ( <div> <Suspense fallback={<div>Loading...</div>}> <LazyComponent /> </Suspense> </div> ); }
In this example, LazyComponent
will only be loaded when it's needed, and while it's loading, the fallback UI will be displayed.
Putting It All Together
These advanced concepts - Context, Portals, Error Boundaries, and Suspense - provide powerful tools for solving common problems in React applications:
- Use Context for managing global state and avoiding prop drilling.
- Use Portals for rendering components outside the normal DOM hierarchy.
- Implement Error Boundaries to gracefully handle errors and improve user experience.
- Leverage Suspense for better handling of asynchronous operations and code splitting.
By mastering these concepts, you'll be well-equipped to build complex, robust React applications that can handle real-world scenarios with ease.
6. Putting It All Together: Best Practices and Next Steps
As we wrap up our journey through the world of React, let's take a moment to consolidate what we've learned and look at some best practices that will help you write clean, efficient, and maintainable React code.
React Best Practices
-
Component Organization:
- Keep components small and focused on a single responsibility.
- Use a consistent file structure for your components (e.g., one component per file).
-
State Management:
- Keep state as local as possible.
- Use Redux or Context API for global state management only when necessary.
-
Performance Optimization:
- Use React.memo for functional components that render often with the same props.
- Implement
shouldComponentUpdate
in class components or use PureComponent. - Use the
useCallback
hook to memoize functions passed as props.
-
Code Splitting:
- Use dynamic imports and React.lazy for code splitting to reduce initial bundle size.
-
Testing:
- Write unit tests for your components using tools like Jest and React Testing Library.
- Implement integration tests to ensure different parts of your app work well together.
-
Styling:
- Consider using CSS-in-JS solutions like styled-components or CSS Modules for scoped styling.
-
Accessibility:
- Always use semantic HTML elements.
- Implement proper ARIA attributes where necessary.
- Ensure your app is keyboard navigable.
Continuous Learning
React and its ecosystem are constantly evolving. Here are some areas you might want to explore next:
-
Server-Side Rendering (SSR): Look into Next.js for building server-rendered React applications.
-
Static Site Generation: Explore Gatsby for building blazing-fast static websites with React.
-
State Management Libraries: While we've covered the Context API, you might want to dive into Redux or MobX for more complex state management scenarios.
-
React Native: If you're interested in mobile development, React Native allows you to build native mobile apps using React.
-
GraphQL: Many React developers are adopting GraphQL (often with Apollo Client) for more efficient data fetching.
-
TypeScript: Consider adding static typing to your React projects with TypeScript for improved developer experience and code quality.
Conclusion
Congratulations! You've now got a solid foundation in React, from the basics of components and JSX, through state management and hooks, to advanced concepts like Context and Error Boundaries.
Remember, becoming proficient in React is a journey. The concepts we've covered here are just the beginning. As you build more projects and dive deeper into the React ecosystem, you'll discover new patterns, tools, and best practices that will help you become an even better React developer.
Don't be afraid to experiment, make mistakes, and learn from them. Join React communities, contribute to open-source projects, and keep building. Every line of code you write is a step forward in your development journey.
Now, armed with this knowledge, it's time to start building! Create that project you've been thinking about, contribute to an open-source React library, or dive into some of the advanced topics we mentioned.
The world of interactive, efficient web apps awaits you. Happy coding, and welcome to the exciting world of React development!
Tags
About the Author
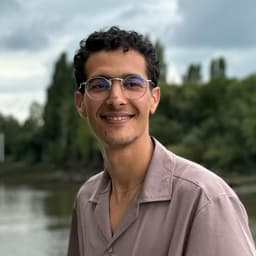
Ahmed Oublihi
A passionate Software Developer and technologist exploring the intersections of code and creativity.